Hotel Booking Chatbot using real APIs
In this tutorial, you will learn how to create your own chatbot using Functionary's function calling feature with some real world APIs. Equipping Functionary to call real world APIs helps you connect Functionary to external systems that provide the most up-to-date and accurate information. Through this tutorial, you will see how easy it is to set up Functionary to call real world APIs and how Functionary is able to plan between different API endpoints towards answering users' queries. All the code provided here can also be found in this Github repository which contains information on how to get the chatbot running in your local machines.
An Application Programming Interface (API) is a kind of mechanism that enables communication between two software systems. Data is sent via requests and responses. In a typical setting, the client application sends the request to the server and the server will send the corresponding response containing the data that fulfills the request back to the client. For example, the road traffic app in your phone is able to send an API request requesting for the traffic at certain areas to the national transport ministry's road traffic monitoring system. The road traffic monitoring system will then send the relevant response back to the mobile app to display to the user.
The request-response nature of APIs works similarly to how functions work (receiving inputs and providing outputs). Furthermore, the above example illustrates that APIs allow for retrieving the most up-to-date and accurate information. Thus, APIs serve perfectly well as effective tools to be used by Functionary in real world applications. In this tutorial, we will demonstrate this ability with a simple hotel booking chatbot.
In this tutorial, you'll build a simple hotel booking chatbot application in Python with Functionary and real APIs from hotel-com-provider in RapidAPI. Your chatbot will be able to provide information about the hotels that users are interested in, and help users search for hotels for their upcoming holidays.
- An understanding of how to run Functionary vLLM server and make API requests to the running server
- Basic skills in Python programming and interacting with APIs
- How to subscribe to and use APIs from RapidAPI
- How to register API Endpoints as tools for Functionary
- How to call Functionary and get function call responses
- How to return the function response to Functionary and respond to the user
- How to create a simple chatbot in command-line interface that iteratively converses with the user and makes function calls
- A machine capable of running inference with a local functionary-small-v2.2 or older model (at least 24GB GPU VRAM)
- A machine with Functionary's dependencies installed
Start a Functionary vLLM server with any functionary model.
Before you can start using any public APIs as tools for Functionary, you need to sign up for a RapidAPI account and subscribe to the APIs that you need.
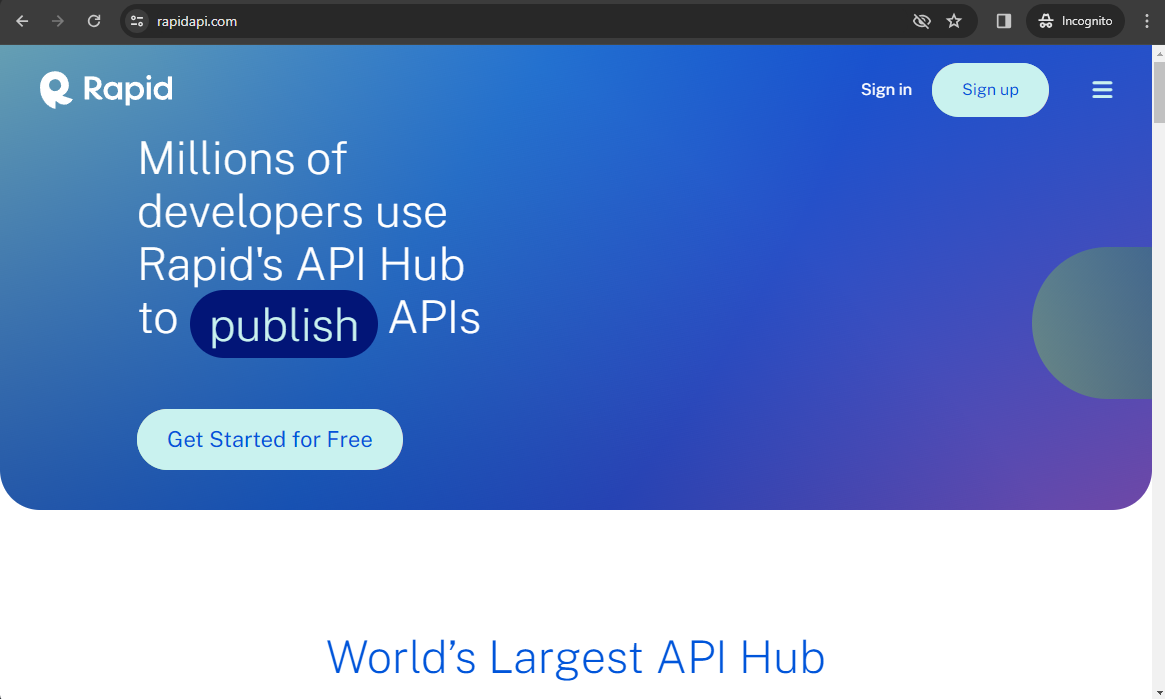
- Click on the "Sign up" button on the top-right hand corner of the RapidAPI homepage as shown above.
- You will be brought to the signing up page where you can choose either to sign up with Google, Github, Facebook or manually.
After logging in, proceed to the RapidAPI Hub page. Thereafter, search for the hotels-com-provider APIs as shown below:
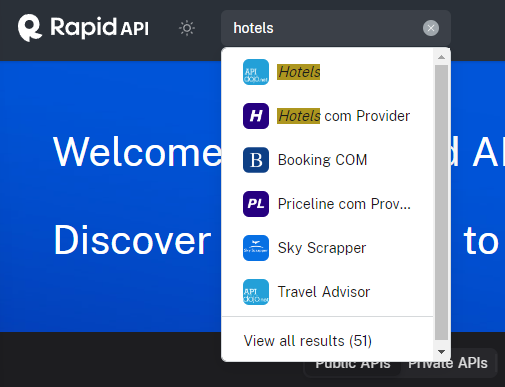
Click on the "Hotels com Provider" option and it will bring you to the API Endpoints page. In this page, click on "Pricing" at the top of page
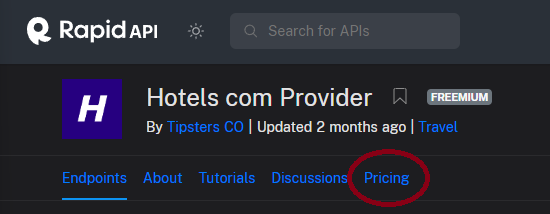
Over at the Pricing page, you are allowed to choose different plans which over different request and rate limits. Since Hotels-com-provider is a freemium type of API, we will go ahead and choose the free Basic plan. It has a request and rate limit of 600/month and 5/second respectively, enough for the purpose of this tutorial.
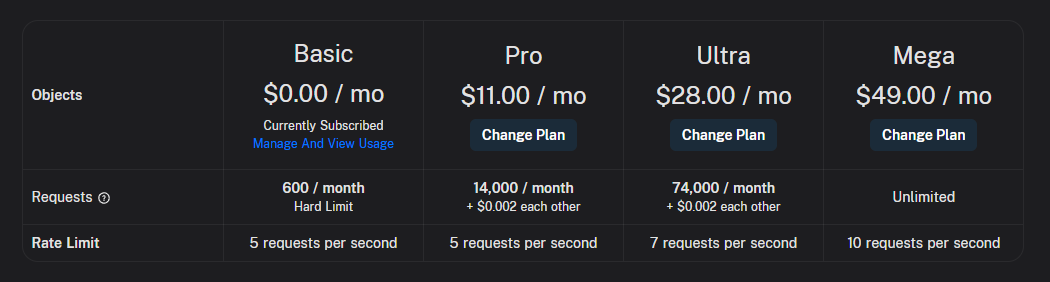
The Hotels com provider APIs provide several endpoints. For this tutorial, we will just use the following endpoints:
- Hotels Search -> Searches hotels by region_id and preferences
- Hotel Details -> Gets the details of a particular hotel
- Regions Search -> Search for region/location/city/hotel by name and returns the respective id (needed by both Hotels Search and Hotel Details)
These endpoints provide the necessary tools for a simple hotel booking chatbot where there are search and information gathering capabilities regarding hotels.
The Functionary OpenAI-compatible vLLM server works with tools defined in OpenAI's format. Thus, we will need to provide the API endpoints in the same tool definition format for Functionary to access. Each tool and its parameters contains the name, description, and various requirements indicated in JSON schema format. Fortunately, RapidAPI provides the details for each API endpoint in the Platform API. For example, lets look at the Hotel Details endpoint on Platform API page:
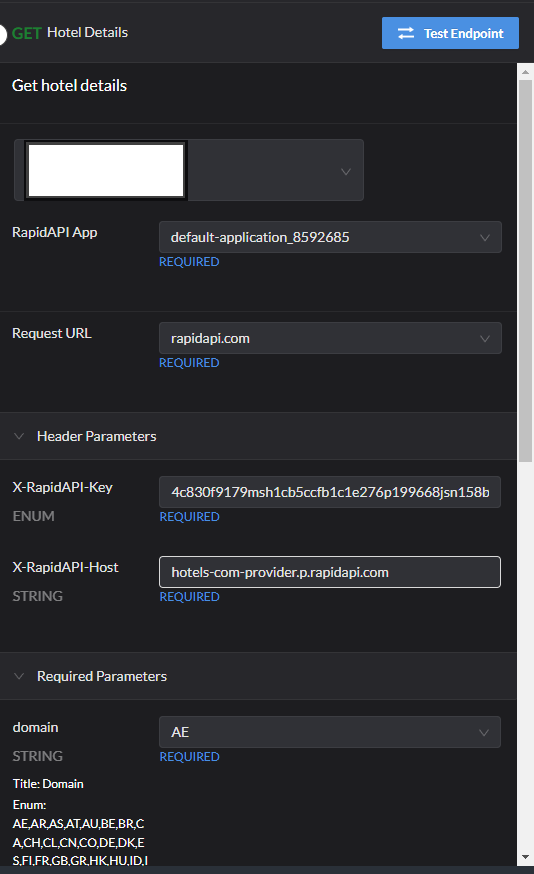
The endpoint description can be converted to tool description while each parameter contains all the necessary information to be converted to the JSON schema format compatible with OpenAI tools. After copying the information over, here's the list of tools:
Note that the "locale" and "domain" parameters have been set to "en_US" and "US" respectively by default while the optional parameters are left out for convenience. Since the regions_search tool is required by the other two tools (region_id and hotel_id respectively), the tool description was modified to reflect this too. This JSON schema definition can also be found in the hotels_api_specification.json file contained in the Github repository of this tutorial.
To use the API-converted tools that we have just created, we can simply load the JSON file containing the tools as a Python List variable and provide the tools for model inference.
Now that you have learnt how to subscribe to the hotel planning API endpoints by Hotels-com-provider and how Functionary call the APIs via generating tool call, we can proceed to the actual task of creating your own hotel planning chatbot. We will first begin by introducing the overall pipeline and important points to note, followed by going through each step in the pipeline. The code for creating the chatbot can be found in this Github repository.
The main variable in the chatbot application is messages. messages is a List variable that tracks the current conversation. Each turn in messages is a Dictionary following the same specification created by OpenAI.
The following flowchart shows the pipeline design of the hotel planning chatbot. We will go in-depth into the various stages in the pipeline in the following subtopics.
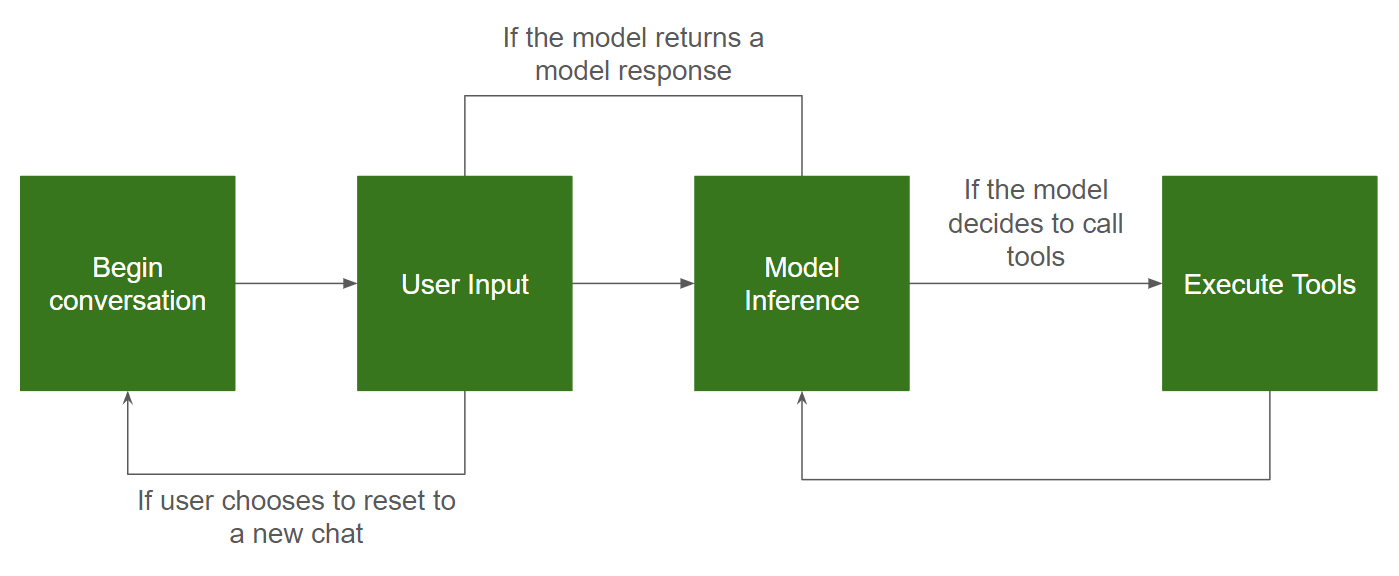
We first begin the conversation. Whenever we reset the conversation, messages will be re-initialized to contain the introductory messages only. The introductory messages consists of a greeting by the user and the intro_msg by the assistant. This is added to prompt-engineer Functionary to be able to identify itself as a hotel planning chatbot.
Once the user input is provided, it will be packaged into a user message with the "content" field being the user query. messages will then be passed to Functionary for inference. We will use the OpenAI API to send requests to the Functionary vLLM server:
Note that after we receive the output message response, we will perform some postprocessing. Specifically, if the response contains tool calls, the infer_model function will output a list of dictionaries containing the tool name and arguments. Otherwise, the function will return the model response in string format if the response is a model response.
If Functionary generates a decision to call tools, we will need to execute them by making API requests to the respective Hotels-com-provider RapidAPI API endpoints. An overview of the process of calling tools and receiving their responses is illustrated in the figure below:
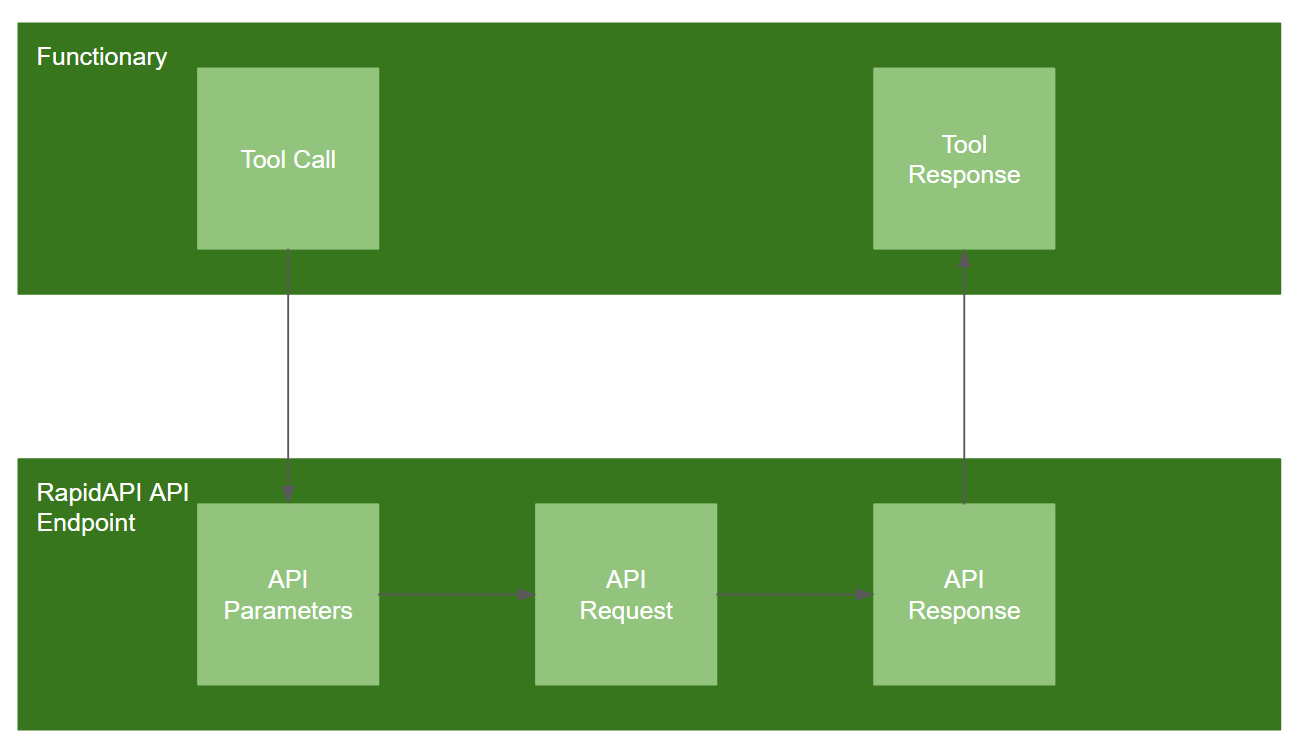
When Functionary decides to make a tool call, it will generate the name of the tool and its arguments. The arguments are also the parameters/inputs for the corresponding API endpoint. With all the information provided, an API request can then be sent to the API endpoint. Once the API endpoint finishes processing the request, it will send the response back to our program. We will then include the API responses into messages as part of the conversation. The following code snippets shows how to call the hotels-com-provider APIs:
First, we need to check if the tool name provided by Functionary is a valid API name. This is just an additional check since with grammar sampling enabled, Functionary will always generate a valid tool name. Thereafter, we will make the request to the endpoint via Python's requests package. RapidAPI's Platform API page has example codes for various languages, including using Python requests package.
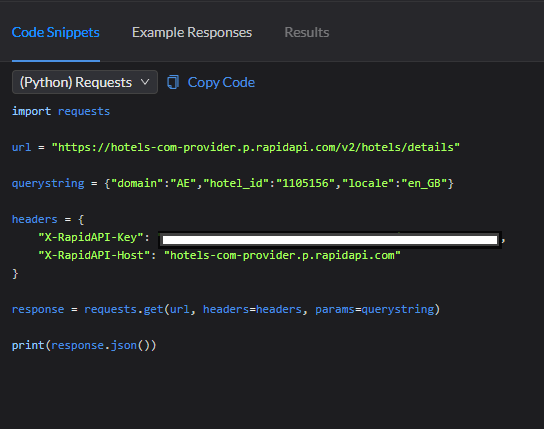
Note that a RapidAPI API key is needed for each request. You can find it in the code snippets section.
Once we have the response, we will do some slight postprocessing before returning the responses to Functionary. If the API is regions_search, we will return either "region_id" or "hotel_id" and "city_id" depending on whether the search query is a region or a hotel. If the API is hotels_search, we will return top-5 search results with each entry containing "name", "price_per_night", "review_score", "hotel_id", etc. If the API is hotel_details, we will return the information of the hotel in "hotel_info".
Congratulations on completing this tutorial and successfully creating your first hotel planning chatbot using Functionary's function calling/tool use capability! We hope you have learnt about how Functionary's function calling feature can be used with real world APIs as tools. Users can ask about hotel recommendations, hotel information, etc. and the system will intelligently fetch the latest data from an external API and respond with an answer.
Functionary's function calling feature is intended to help you with setting up your own chatbot applications. It can call functions intelligently, extract parameters as deterministically as possible, and performs analysis and content creation. You just need to provide it with your desired APIs and it will handle the rest towards creating useful and helpful responses to users. Feel free to try out other APIs in your own pipeline and explore the capabilities of Functionary.